Create multi-step forms and transfer form field values between Webflow pages. Using JavaScript, pass values via URL query parameters and auto-populate form fields on the redirect page.
Webflow: Pass form values to a redirect page and populate another form fields
Case
- Call a user to leave their email (subscribe to the newsletter),
- capture email and redirect to a Sign Up page on submission,
- and suggest to create an account with password at the Sign Up page with the email value prefilled to the login field.
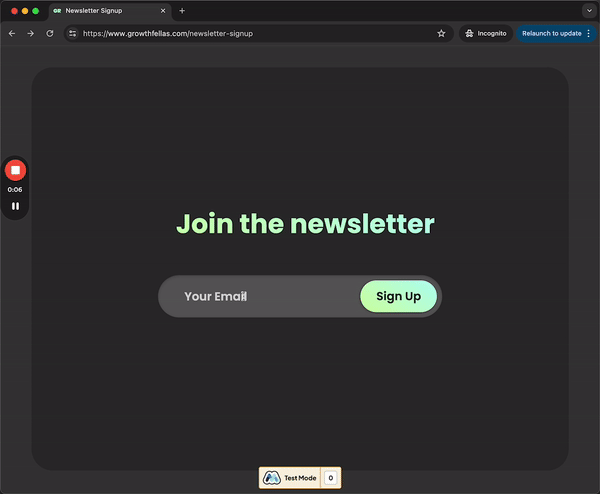
Method
- add the email field value to the redirect URL query string parameters, ../destination-page?email={email};
- retrieve the email parameter from the URL and populate an input field with its value at the destination page.
Set up a form at the source page
Step 1: Create the form
- Use the standard Webflow form:
- Form block ID: newsletterform;
- Method: POST;
- Redirect URL: leave empty (we will asign it via custom code);
- Action: leave empty.
- Create a field:
- ID: emailinput
- Type: Email
Step 2: Capture email field value and set up redirect using JavaScript
At the source page go to Settings and insert the following code to the </body> tag.
<script>
document.getElementById("newsletterform").addEventListener("submit", function(e){
e.preventDefault();
// Get the value from the email input field
var emailinputValue = document.getElementById("emailinput").value;
// Redirect to the destination page with the email as a URL parameter
window.location = "destinationpage?email=" + emailinputValue;
});
</script>
, where:
- e.preventDefault(); — prevents the default Webflow form submission action.
- var emailinputValue — Captures the value of the email input field.
- window.location — redirects the user to the destinationpage with the captured email value as a URL parameter.
Step 3: Extract email from URL parameter and populate the Log In form field
On the destination page, ensure the email input field has an ID to receive the value, such as email.
<script>
window.addEventListener('load', function() {
// Get the full URL
var url_string = window.location.href;
var url = new URL(url_string);
// Extract the email parameter from the URL
var emailinput = url.searchParams.get("email");
// Set the form field with the extracted email value
document.getElementById('email').value = emailinput;
});
</script>
, where:
- window.addEventListener('load', function() {...}); — Ensures the script runs after the page has fully loaded.
- var url_string and var url — retrieve the current page URL.
- url.searchParams.get("email") — extract the email value from the URL parameter.
- document.getElementById('email').value = emailinput; — populates the email field with the extracted email value.
With this implementation, the email entered in the newsletter form on the main page will be passed to the form on the destination page in URL string parameters and pre-filled to the email field at a sign up form.
Related hacks
MNNGFUL Inc.
mjei.me
Log In or Sign Up above to join the conversation.
Log In or Sign Up to join the conversation.
Join the discussion
0 comments